A few days ago, we talked about the ngOnChanges
lifecycle hook. We saw that every time an @Input()
changes, Angular will trigger ngOnChanges
. ngOnInit
is similar but runs only once; after all @Input()
receive their initial value. The official documentation puts it like this:
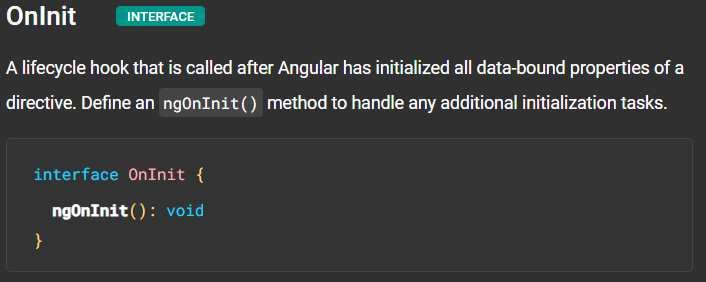
The above definition might surprise you because there is a widespread belief that the purpose of ngOnInit is to run all initialization tasks, even though the class constructor runs before ngOnInit
, as explained a few months ago in this entry: ngOnInit vs. constructor in Angular.
As a result, using ngOnInit
is only 100% justified when you want to run some code that needs the value(s) of @Input()
properties of your component/directive. In other scenarios, you can use a constructor to run your initialization code sooner.