Modern Javascript developers are used to the following syntax to create a copy of an object:
let copy = {...object};
Code language: JavaScript (javascript)
While this approach works fine, it creates a shallow copy of the object, not a fully-fledged clone that also duplicates nested objects and arrays.
As a result, Javascript developers have learned another trick to perform a deep copy:
let copy = JSON.parse(JSON.stringify(object));
Code language: JavaScript (javascript)
The idea of this approach is to turn an object into a JSON string before parsing that string back into a brand-new object. It works, but it’s not elegant and looks like a hack.
As a result, a better approach has been added to the Javascript language: the structuredClone
function. It’s a syntax improvement that creates deep clones efficiently:
let copy = structuredClone(object);
Code language: JavaScript (javascript)
The function is supported across all recent major browsers as shown on Can I use:
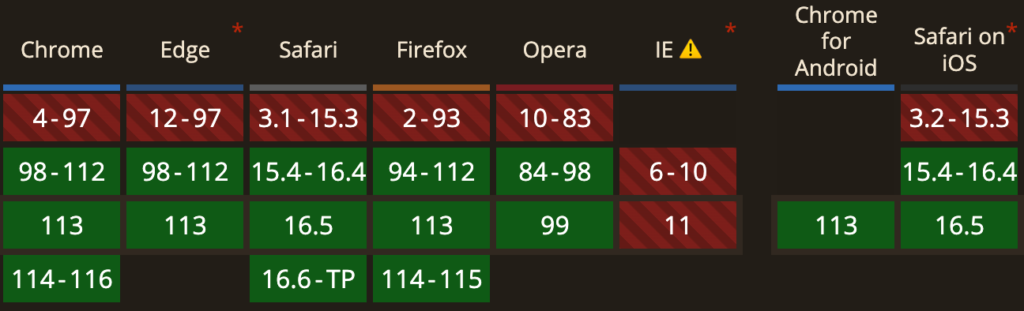
If you need to support another browser, there is a polyfill implementation in core.js.