At this point, it is safe to assume that most Angular developers know about the following syntax. That’s how we subscribe to an Observable to receive its data:

If you’ve been reading this newsletter for long enough, you already know that there are better ways to subscribe to Observables and that there are no excuses for not using these techniques.
That said, it’s always good to know about all our available options. So here is another possible syntax:
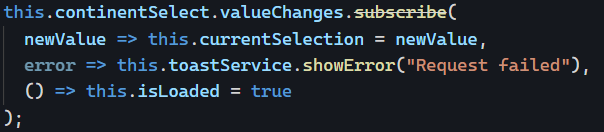
The above syntax registers three different callback functions:
- The first one is the same as in our first example – this is where the Observable data goes.
- The second one is an error handler. Any exception thrown by the Observable would get caught by that callback.
- The third callback function is invoked when the Observable completes, which means it won’t emit any more value.
Note that while that syntax still works (for now), it has been deprecated in favor of a more explicit syntax, where an object is passed. Each callback is attached to a named property, making the purpose of these callbacks more obvious:
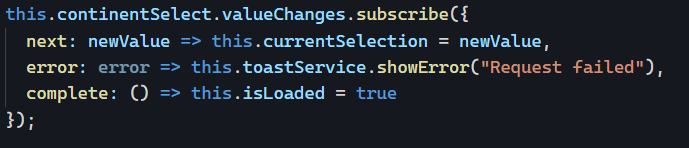