A while back, I wrote about how ngrxLet
is an improved version of the async pipe. I also covered how to use skeleton loaders with Angular.
Today, let’s look at how we can use these two tools to display a “loading” template while an observable is waiting for data.
As a reminder, here is how ngrxLet
can be used to track different observable events:
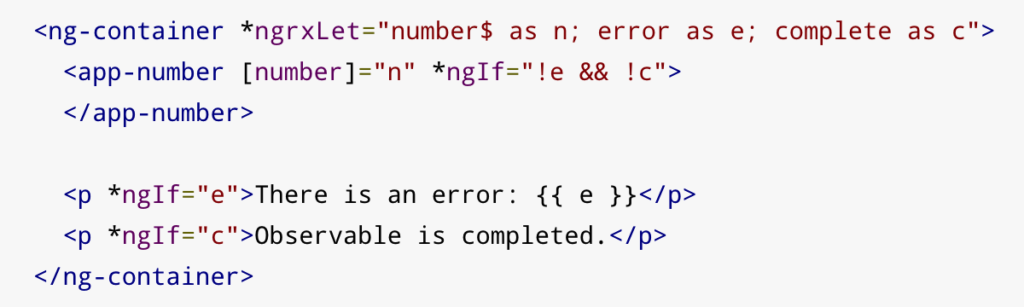
In the above code snippet, we receive the values emitted by the number$
observable in a variable n
. We would receive any error in a variable e
. We would receive the completion status (true
or false
) in a variable c
.
Here is how we can pass a custom loading template to ngrxLet
using a template reference variable:
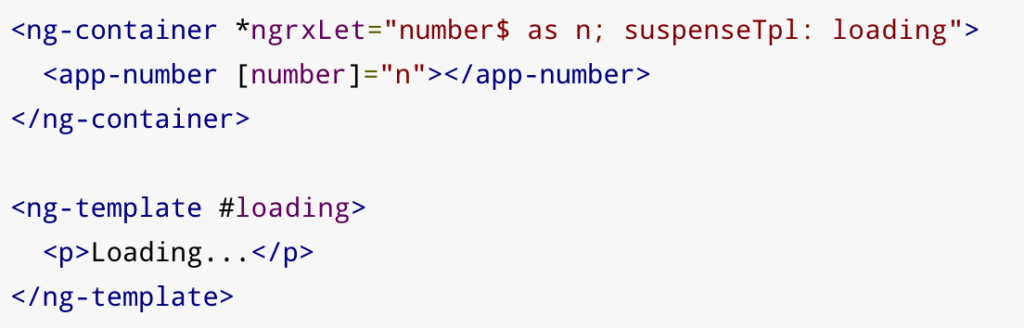
Of course, the loading template can be customized with anything you want, including a skeleton loader or an animated gif image. That feature is called a suspense template, and an observable is in a suspense state until it emits its first event (next
, error
, or complete
as covered here).