Recently, during an Angular Accelerator coaching call, my client asked what is the point of using FormGroup
. I then realized I had never written about FormGroup
, so here is a post to fix that.
When using reactive forms, we always have at least one FormGroup
, which happens to be the entire form. This doesn’t mean that Form Groups are only designed for that purpose: A complex form can be divided into multiple groups. For instance, if we have a form where a user is supposed to enter their name, address, birth date, etc., we could group some of these controls into FormGroups
.
What do we get out of that? A few different things:
- Form groups aggregate the validation state of all individual controls into a group state. If an address is made of 4 controls (street, city, zip code, country), then the form group for these 4 controls will be invalid when any controls are invalid, touched when any control is touched, etc.
- Form groups aggregate actions on all individual controls within the group. Want to disable address entry? Instead of disabling all 4 controls one by one, you can do
addressGroup.disable()
, which is a lot less error-prone (you can’t forget one of these controls) - Form groups store the value of all controls as a single object. In my example with 4 controls for an address, accessing
addressGroup.getValue()
would return:
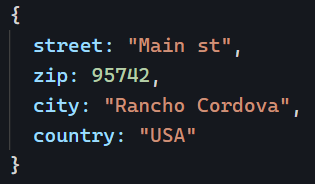
So, the main benefit is to have grouped actions and status updates for a bunch of form controls. Let’s take another example with actual code (you can find the full code base on Stackblitz here):
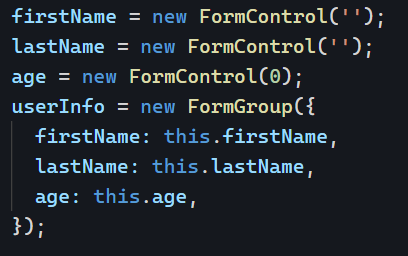
If I use the following expression in my template to see the value of my userInfo
, here’s what I get:

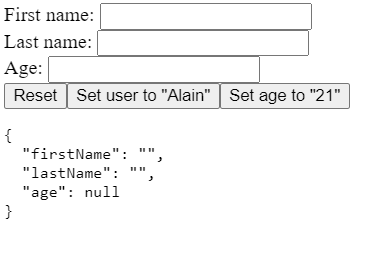
As you can see, my form group creates an object that updates when individual control values change. Perfect! Now, let’s click a button that sets the form group value to an object:


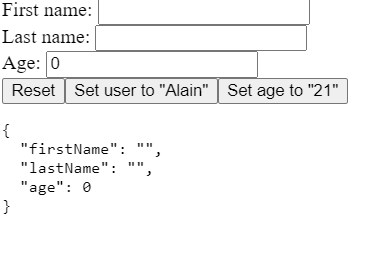
Now, I can use multiple values with one line of code. There is no need to call setValue()
on all controls one by one. That’s cool. What if I want to apply a “diff” to my group and not set the entire thing? Say I wanted to be younger but not change my name or anything else:

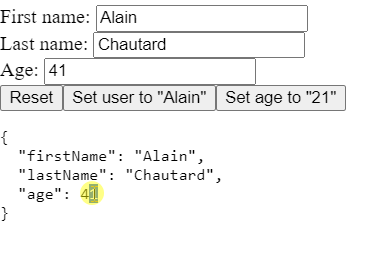
Now, I can “patch” my form group with some localized changes, and I don’t need to worry about the other values of the controls of that group. That’s the magic of formGroup.patchValue()
.
You can also reset the entire group with formGroup.reset()
, add or remove controls and validators, and more. The full API is here. That’s the power of FormGroups
in action!